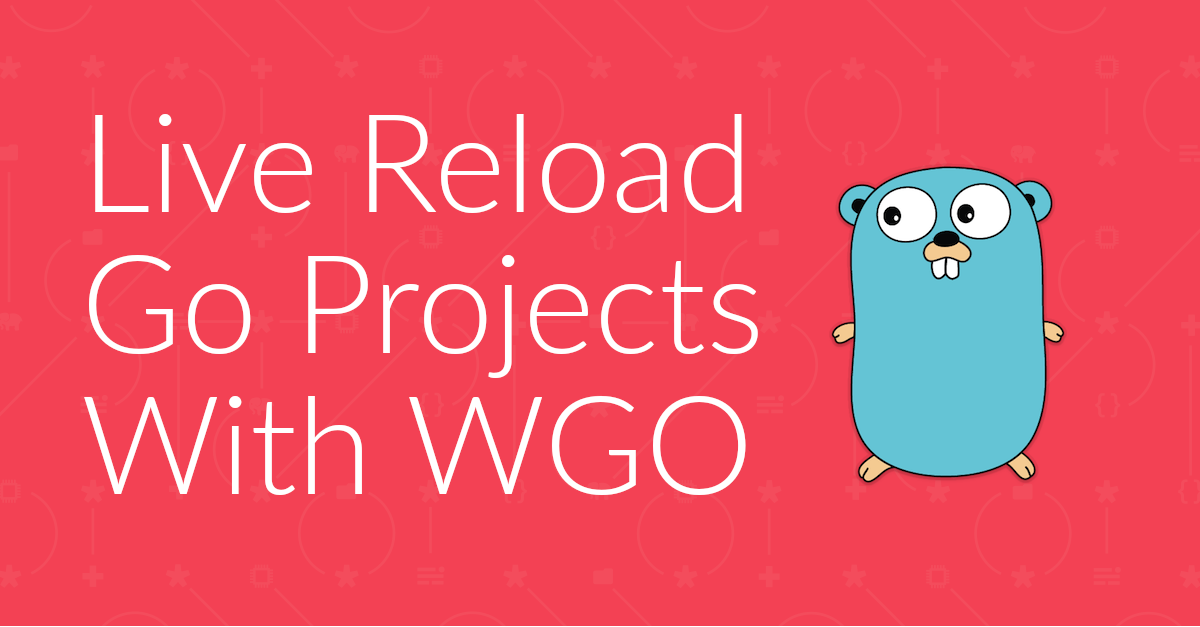
Live Reload Go Projects with wgo
Building web apps in Go is extremely rewarding. However, as Go’s a compiled language, to see changes, you need to restart the app. Needless to say that’s quite tedious! With live reloading, it doesn’t need to be.
As software developers you should use coding standards. In today’s post, learn how to enforce the PSR-1 and PSR-2 coding standards with PHPStorm.
As a software developer, one of the key things that you want to should do is use a coding standard when writing code.
We’re all imperfect; we all have a wide variety of important things which need doing on a daily basis; we all have things competing with us for equal attention.
But that can’t be an excuse to drop the ball and resort to the perennial excuse of: “I don’t have time”. Good coding standards can save us time and effort. I know, I know:
Matt, you don’t understand my situation, I’m flat for time because…
The thing is, I don’t need to understand your situation and you don’t need to understand mine. We’re all busy. Let’s accept that and move on.
You can’t pay lip service to wanting to do better, but then do nothing about it. The best thing is, by enforcing coding standards, you don’t need – and it’s not hard to do so.
I believe we should all pick, and stick to, a coding standard which will help us to develop better, more maintainable, easier to read and debug code.
At first it’ll probably be annoying and frustrating. But every new endeavour can be. But most of them, the worthwhile ones at least, with time, become second nature, something you don’t need to think about.
In today’s post, I want to quickly show you how to enforce a coding standard, specifically PSR-1 and PSR-2, when developing with my favourite PHP editor – PHPStorm.
If you’re not using PHPStorm, I strongly encourage you to do so. It’s nothing short of amazing. What’s more, given that it’s written in Java, the speed and responsiveness, especially when combined with the amount of features it offers, really is something to behold.
PSR-1 is the basic coding standard for PHP and PSR-2 is the coding style guide. PSR-1 covers such requirements as:
PSR-2 covers such requirements as:
Together, they help ensure that code is well organised and follows standard coding conventions. Here’s an example of code which conforms to both standards:
namespace App;
use FooInterface;
use BarClass as Bar;
use OtherVendorOtherPackageBazClass;
class Foo extends Bar implements FooInterface
{
public function sampleFunction($a, $b = null)
{
if ($a === $b) {
bar();
} elseif ($a > $b) {
$foo->bar($arg1);
} else {
BazClass::bar($arg2, $arg3);
}
}
final public static function bar()
{
// method body
}
}
PHPStorm, IMHO, is the best editor available for PHP. Whilst not cheap in a dollar sense (€89 for an individual developer or €179 for a commercial developer, per/year), it’s an investment well worth making for the return you get.
Here’s a short selection of the features on offer:
It’s just amazing as to all the things it can do. Definitely give the trial version a go if you’ve not already.
Now let’s look at how to enforce PSR-1 and 2 when using PHPStorm. From application preferences, either scroll down to the Code Style section, then choose PHP, or type PHP in the search box, which will filter the options available for you and then click PHP under Code Style, as in the screenshot above.
This will display the Code Style preferences pane. On the right-hand side, near the top of the pane, in blue you’ll see the link Set from….
Clicking that will display a drop-down menu, with 2 choices: Language and Predefined Style. Mouse-over Predefined Style and there you’ll see a sub-menu appear with the 6 code style options supported by PHPStorm, as in the screenshot below.
Click PSR1/PSR2, then click OK in the bottom right of the preferences window. You don’t need to restart PHPStorm as the setting change applies immediately. Depending on the speed of your computer, it may take a minute or two, at most, for PHPStorm to update the code windows to reflect the change.
From now on, when you’re developing, if you don’t conform to the standard, you’ll see a notification appear to let you know what you need to change to conform to the standard.
So that’s how you set and enforce PSR-1 and 2 in PHPStorm. I strongly encourage you to do so. If you’re using another standard, then enforce that one instead. But whichever way, make sure you’re enforcing a standard, even if for no other reason than to help yourself grow as a developer.
Building web apps in Go is extremely rewarding. However, as Go’s a compiled language, to see changes, you need to restart the app. Needless to say that’s quite tedious! With live reloading, it doesn’t need to be.
What is go mod tidy and why you would use it? In this short tutorial you’ll get a brief introduction to what it is and see the essentials of how to use it.
In versions of Xdebug before version 3 setting up step debugging for code inside Docker containers has often been challenging to say the least. However, in version 3 it’s become almost trivial. In this short tutorial, I’ll step you through what you need to do, regardless of the (supported) text editor or IDE you’re using.
PhpStorm offers so much functionality. From syntax highlighting to Docker integration, it’s an extremely comprehensive tool. However, have you ever thought of using it to run your unit tests? In this article, I step you through running tests, from an entire suite to an individual test.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus